OBJETIVOS
El objetivo de esta práctica es explicar en detalle y de una manera clara y sencilla el uso de la librería gráfica GFX de Adafruit para gestionar el funcionamiento de una pantalla OLED a través del procesador ESP8266.
La librería gráfica nos permitirá, de una manera simple, dibujar puntos, líneas, triángulos, rectángulos y circunferencias o insertar texto o imágenes en nuestra pantalla. En las explicaciones podremos encontrar ejemplos detallados de su uso.
En este caso se utilizará una placa NodeMCU que lleva instalado dicho procesador y una pantalla OLED monocroma de 128×64 pixels con conexión I2C, sin embargo, dado el carácter general de las explicaciones, esta práctica también sera útil para conectar y hacer funcionar otros modelos de placas y/o pantallas, siempre y cuando se tengan en cuenta sus características técnicas específicas.
MATERIAL NECESARIO
CONCEPTOS BÁSICOS
INSTALACIÓN DE LAS LIBRERÍAS
La librería gráfica GFX de Adafruit se instala desde el IDE de Arduino seleccionando:
Programa → Incluir librería→ Gestionar Librerías → Adafruit GFX Library
Esta librería siempre trabaja en común con una segunda librería que es específica del modelo de pantalla a utilizar, que también se puede instalar desde el Gestor de Librerías del IDE de Arduino. En la siguiente tabla hay una relación de todos los modelos de pantallas que funcionan con la librería gráfica GFX y la librería específica que le corresponde a cada pantalla:
SISTEMA DE COORDENADAS
Los puntos de la pantalla –pixels– se referencian en un sistema de coordenadas cartesianas. El origen (0,0) está en la esquina superior izquierda y su eje horizontal es es el X y el eje vertical el Y.
Este sistema nos permitirá situar los objetos que dibujemos en su posición correcta y con las dimensiones que deseemos.
El tamaño de los pixels específico de cada pantalla. Si queremos pasar a otra unidad de medida como milímetros deberemos hacer los cálculos correspondientes, utilizando los datos número de pixels y dimensiones de las especificaciones técnicas.
COLOR
- En las PANTALLAS MONOCROMAS los colores se especifican simplemente como 1 (activo) ó 0 (inactivo). La semántica de activo/inactivo es específica para el tipo de pantalla. En las pantallas luminosas, como las OLED un pixel activo se ilumina, mientras que en las reflectantes, como las LCD, un pixel activo se oscurece. Un píxel inactivo representa el estado de fondo predeterminado para una pantalla recién inicializada -antes de realizar cambios-.
- En las PANTALLAS DE COLOR los colores se representan con un valor de 16 bits -sin signo-. Algunas pantallas pueden ser físicamente capaces de más o menos bits, pero la librería funciona con 16 bits. A continuación hay una lista de los colores primarios y secundarios más comunes, que puede ser útil para incluir en el sketch, aunque por supuesto, se pueden elegir cualquiera de 65.536 colores diferentes.
// Definición de colores #define BLACK 0x0000 //Negro #define BLUE 0x001F //Azul #define RED 0xF800 //Rojo #define GREEN 0x07E0 //Verde #define CYAN 0x07FF //Cian -azul claro- #define MAGENTA 0xF81F //Magenta -fucsia- #define YELLOW 0xFFE0 //Amarillo #define WHITE 0xFFFF //Blanco
- En los PANELES LED los colores se representan con un valor del 0 al 7 por cada color de la composición RGB (rojo-verde-azul) -3 bits-, por lo que el panel tiene una posibilidad de 8x8x8=512 colores.
FUNCIONES GRÁFICAS -FORMAS GEOMÉTRICAS BÁSICAS, IMÁGENES Y TEXTO-
PUNTOS -PIXELS- |
void drawPixel(x0, y0, color) |
Se dibuja un punto con la función void drawPixel(), indicando sus coordenadas y el color. Ejemplos: //Librerías SSD1306, SSD1331, Graphic VFD, PCD8544 y HX8340B display.drawPixel(17,11,WHITE); //Librerías ST7735 e ILI9341_t3 tft.drawPixel(17,11,0x001F); //Librería RGB Matrix Panel matrix.drawPixel(17,11,matrix.Color333(7,0,0)); |
LÍNEAS |
void drawLine( x0, y0, x1, y1, color) |
Se dibuja una línea con la función void drawLine(), indicando las coordenadas de sus extremos y el color. Ejemplos: //Librerías SSD1306, SSD1331, Graphic VFD, PCD8544 y HX8340B display.drawLine(4,5,18,9,WHITE); //Librerías ST7735 e ILI9341_t3 tft.drawLine(4,5,18,9,1,0x07E0); //Librería RGB Matrix Panel matrix.drawLine(4,5,18,9,matrix.Color(0,7,0)); |
TRIÁNGULOS Y TRIÁNGULOS RELLENOS |
void drawTriangle( x0, y0, x1, y1, x2, y2, color) |
Se dibuja un triángulo con la función void drawTriangle(), indicando las coordenadas de sus tres vértices y el color. Ejemplos: //Librerías SSD1306, SSD1331, Graphic VFD, PCD8544 y HX8340B display.drawTriangle(5,3,20,10,2,14,WHITE); //Librerías ST7735 e ILI9341_t3 tft.drawTriangle(5,3,20,10,2,14,0xF800); //Librería RGB Matrix Panel matrix.drawLine(5,3,20,10,2,14,matrix.Color333(7,0,0)); |
void fillTriangle( x0, y0, x1, y1, x2, y2, color) |
Se dibuja un triángulo relleno con la función void fillTriangle(), indicando las coordenadas de sus tres vértices y el color. Ejemplos: //Librerías SSD1306, SSD1331, Graphic VCD, PCD8544 y HX8340B display.fillTriangle(5,3,20,10,2,14,WHITE); //Librerías ST7735 e ILI9341_t3 tft.fillTriangle(5,3,20,10,2,14,0xF800); //Librería RGB Matrix Panel matrix.fillTriangle(5,3,20,10,2,14,matrix.Color333(7,0,0)); |
RECTÁNGULOS Y RECTÁNGULOS RELLENOS |
void drawRect( x0, y0, ancho, alto, color) |
Se dibuja un rectángulo con la función void drawRect(), indicando las coordenadas de su esquina superior izquierda, anchura, altura y color. Ejemplos: //Librerías SSD1306, SSD1331, Graphic VFD, PCD8544 y HX8340B display.drawRect(5,3,14,12,WHITE); //Librerías ST7735 e ILI9341_t3 tft.drawRect(5,3,14,12,0xF81F); //Librería RGB Matrix Panel matrix.drawRect(5,3,14,12,matrix.Color333(7,0,7)); |
void fillRect( x0, y0, ancho, alto, color) |
Se dibuja un rectángulo relleno con la función void fillRect(), indicando las coordenadas de su esquina superior izquierda, anchura, altura y color. Ejemplos: //Librerías SSD1306, SSD1331, Graphic VFD, PCD8544 y HX8340B display.fillRect(5,3,14,12,WHITE); //Librerías ST7735 e ILI9341_t3 tft.fillRect(5,3,14,12,0xF81F); //Librería RGB Matrix Panel matrix.fillRect(5,3,14,12,matrix.Color333(7,0,7)); |
RECTÁNGULOS REDONDEADOS Y RECTÁNGULOS REDONDEADOS RELLENOS |
void drawRoundRect( x0, y0, ancho, alto, radio, color) |
Se dibuja un rectángulo redondeado con la función void drawRoundRect(), indicando las coordenadas de su esquina superior izquierda, anchura, altura, radio de redondeo y color. Ejemplos: //Librerías SSD1306, SSD1331, Graphic VFD, PCD8544 y HX8340B display.drawRoundRect(2,1,17,14,WHITE); //Librerías ST7735 e ILI9341_t3 tft.drawRoundRect(2,1,17,14,0x0000); //Librería RGB Matrix Panel matrix.drawRoundRect(2,1,17,14,matrix.Color333(0,0,0)); |
void fillRoundRect( x0, y0, ancho, alto, radio, color) |
Se dibuja un rectángulo relleno redondeado con la función void fillRoundRect(), indicando las coordenadas de su esquina superior izquierda, anchura, altura, radio de redondeo y color. Ejemplos: //Librerías SSD1306, SSD1331, Graphic VFD, PCD8544 y HX8340B display.fillRoundRect(2,1,17,14,WHITE); //Librerías ST7735 e ILI9341_t3 tft.fillRoundRect(2,1,17,14,0x0000); //Librería RGB Matrix Panel matrix.fillRoundRect(2,1,17,14,matrix.Color333(0,0,0)); |
CIRCUNFERENCIAS Y CÍRCULOS |
void drawCircle( x0, y0, radio, color) |
Se dibuja una circunferencia con la función void drawCircle(), indicando las coordenadas de su centro, radio y color. Ejemplos: //Librerías SSD1306, SSD1331, Graphic VFD, PCD8544 y HX8340B display.drawCircle(11,8,8,WHITE); //Librerías ST7735 e ILI9341_t3 tft.drawCircle(11,8,8,0x07FF); //Librería RGB Matrix Panel matrix.drawCircle(11,8,8,matrix.Color333(0,7,7));
|
void fillCircle( x0, y0, radio, color) |
Se dibuja un círculo con la función void fillCircle(), indicando las coordenadas de su centro, radio y color. Ejemplos: //Librerías SSD1306, SSD1331, Graphic VFD, PCD8544 y HX8340B display.fillCircle(11,8,8,WHITE); //Librerías ST7735 e ILI9341_t3 tft.fillCircle(11,8,8,0x07FF); //Librería RGB Matrix Panel matrix.fillCircle(11,8,8,matrix.Color(0,7,7)); |
IMÁGENES -BITMAPS- |
void drawBitmap( x0, y0, nombre, ancho, alto, color) |
Se dibuja una imagen en la pantalla con la función void drawBitmap(), indicando las coordenadas de su esquina superior derecha, nombre de la imagen, anchura y altura que debe ocupar en la pantalla y color (las imágenes tendrán un único color). La construcción del bitmap (mapa de bits) de la imagen es sumamente sencilla utilizando la aplicación IMAGE2CPP. Esta aplicación nos permitirá adicionalmente generar el código para que el array (lista de datos), que la contiene se almacene en la memoria Flash del procesador, mediante el modificador de variable PROGMEM. Al utilizar la memoria Flash, reservamos la memoria SRAM para almacenar los datos de ejecución del programa, optimizando su ejecución. Hagamos una prueba generando el array de datos de la siguiente imagen: |
![]() |
Paso 1 |
![]() |
|
Paso 2 |
![]() |
En la previsualización (preview) vemos como quedará la imagen en la pantalla. |
Paso 3 |
![]() |
Este es código que obtenemos como resultado, para añadir al programa y poder visualizar la imagen: const unsigned char Toro [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0xff, 0xff, 0xe0, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x70, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0x18, 0x00, 0x00, 0x00, 0x1c, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x18, 0x00, 0x00, 0x00, 0x7d, 0xbf, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x18, 0x00, 0x00, 0x00, 0xfd, 0xbf, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x1c, 0x00, 0x00, 0x00, 0xfd, 0xdf, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x1c, 0x00, 0x00, 0x07, 0xfd, 0xdf, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x1c, 0x00, 0x00, 0x0f, 0xfd, 0xdf, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x1c, 0x00, 0x00, 0x0f, 0xff, 0xef, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf4, 0x00, 0x00, 0x1e, 0x00, 0x00, 0x3f, 0xff, 0xef, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf7, 0xc0, 0x00, 0x1e, 0x00, 0x00, 0x3f, 0xfb, 0xef, 0xff, 0xf3, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf7, 0xf8, 0x00, 0x1e, 0x00, 0x00, 0xff, 0xfb, 0xef, 0xff, 0xfb, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf7, 0xfc, 0x00, 0x1f, 0xe1, 0xfe, 0x7f, 0xfb, 0xef, 0xff, 0xfd, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf7, 0xc4, 0x00, 0x1f, 0xff, 0xff, 0xbf, 0xfb, 0xef, 0xff, 0xfd, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf0, 0x7c, 0x00, 0x1f, 0xff, 0xff, 0xc7, 0xf7, 0xef, 0xff, 0xfe, 0xff, 0xff, 0xff, 0xbf, 0xff, 0xf7, 0xe4, 0x00, 0x0f, 0xff, 0xff, 0xf9, 0xe7, 0xef, 0xff, 0xfe, 0xff, 0xff, 0xff, 0xbf, 0xff, 0xf7, 0xfc, 0x00, 0x07, 0xff, 0xff, 0xfc, 0x1f, 0xdf, 0xff, 0xfe, 0xff, 0xff, 0xff, 0xbf, 0xff, 0xef, 0xdc, 0x00, 0x03, 0xbf, 0xff, 0xfb, 0xff, 0xc7, 0xff, 0xff, 0x7f, 0xff, 0xff, 0xbf, 0xff, 0xef, 0xbc, 0x00, 0x01, 0xbf, 0xff, 0xff, 0xff, 0xb7, 0xff, 0xff, 0x7f, 0xff, 0xff, 0xbf, 0xff, 0xef, 0x3c, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xfe, 0x77, 0xff, 0xff, 0x7f, 0xff, 0xff, 0xbf, 0xff, 0xef, 0x3c, 0x00, 0x01, 0xff, 0xff, 0xff, 0xfd, 0xf7, 0xff, 0xff, 0x7f, 0xff, 0xff, 0xbf, 0xff, 0xef, 0x3c, 0x00, 0x03, 0xff, 0xff, 0xff, 0xfb, 0xef, 0xff, 0xff, 0x7f, 0xff, 0xff, 0x7f, 0xff, 0xf7, 0x3c, 0x00, 0x03, 0xff, 0xff, 0xff, 0xe7, 0xcf, 0xff, 0xff, 0x7f, 0xff, 0xff, 0x7f, 0xff, 0xf7, 0x3c, 0x00, 0x03, 0xff, 0xff, 0xff, 0x1f, 0xdf, 0xff, 0xff, 0x7f, 0xff, 0xff, 0x7f, 0xff, 0xf7, 0xbc, 0x00, 0x03, 0xff, 0xff, 0xff, 0xff, 0x9f, 0xff, 0xff, 0x7f, 0xff, 0xff, 0x7f, 0xff, 0xe3, 0xdc, 0x00, 0x03, 0xff, 0xff, 0xff, 0xfe, 0x3f, 0xfc, 0xff, 0x7f, 0xff, 0xfe, 0xff, 0xff, 0xe1, 0xe4, 0x00, 0x03, 0xff, 0xfc, 0xff, 0x8c, 0x7f, 0xf1, 0x3f, 0x7f, 0xff, 0xfe, 0xff, 0xff, 0xc1, 0xfc, 0x00, 0x01, 0xff, 0xf0, 0xff, 0x81, 0xff, 0xff, 0xcf, 0xff, 0xff, 0xff, 0xff, 0xff, 0xc0, 0xfe, 0x00, 0x01, 0xff, 0xf3, 0xff, 0x9f, 0xff, 0xff, 0xcf, 0xff, 0xff, 0xef, 0xff, 0xff, 0x80, 0x7e, 0x00, 0x03, 0xff, 0xff, 0xff, 0x9f, 0xff, 0xff, 0xf6, 0xff, 0xff, 0xdf, 0xff, 0xff, 0x80, 0x3e, 0x00, 0x03, 0xff, 0xff, 0xff, 0x9f, 0xff, 0xff, 0xf7, 0xff, 0xff, 0xbf, 0xff, 0xfe, 0x00, 0x1c, 0x00, 0x03, 0xff, 0xff, 0xff, 0x9f, 0xff, 0xff, 0xf7, 0xff, 0xff, 0x7f, 0x7f, 0xfe, 0x00, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0x1f, 0xff, 0xff, 0xf7, 0xff, 0xf8, 0xff, 0x3f, 0xfc, 0x00, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0x7f, 0xff, 0xff, 0xf7, 0xff, 0xe7, 0x7e, 0x0f, 0xfc, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xff, 0xff, 0x7f, 0xff, 0xff, 0xf7, 0xff, 0xcf, 0x80, 0x07, 0xfc, 0x00, 0x00, 0x00, 0x7f, 0x9f, 0xff, 0xfe, 0x7f, 0xff, 0xff, 0xf7, 0xfc, 0x3f, 0xe0, 0x07, 0xfc, 0x00, 0x00, 0x00, 0x7e, 0x37, 0xff, 0xfe, 0xff, 0xff, 0xff, 0xcf, 0xe2, 0x7f, 0x80, 0x07, 0xfc, 0x00, 0x00, 0x00, 0xfe, 0x7e, 0x1f, 0xfd, 0xff, 0xff, 0xff, 0xb8, 0x1d, 0xfe, 0x00, 0x0f, 0xfc, 0x00, 0x00, 0x00, 0xfe, 0x3f, 0xdf, 0xf9, 0xff, 0xff, 0xff, 0x77, 0xfb, 0xf8, 0x00, 0x0f, 0xf8, 0x00, 0x00, 0x00, 0x7f, 0xdf, 0xef, 0xf3, 0xff, 0xff, 0xfe, 0xff, 0xf7, 0xe0, 0x00, 0x0f, 0xf8, 0x00, 0x00, 0x00, 0x7f, 0xfe, 0x6f, 0xc7, 0xff, 0xff, 0xf0, 0x7f, 0xef, 0xc0, 0x00, 0x0f, 0xf0, 0x00, 0x00, 0x00, 0x7f, 0xf9, 0xef, 0x8f, 0xff, 0xff, 0xe0, 0x3f, 0xee, 0x00, 0x00, 0x0f, 0xe0, 0x00, 0x00, 0x00, 0x0f, 0x87, 0xee, 0x7d, 0xff, 0xff, 0xc0, 0x0f, 0x9c, 0x00, 0x00, 0x3f, 0x80, 0x00, 0x00, 0x00, 0x0f, 0xff, 0x00, 0xfe, 0xff, 0xff, 0x80, 0x7f, 0x7c, 0x00, 0x00, 0x3f, 0x80, 0x00, 0x00, 0x00, 0x68, 0x3e, 0x00, 0xff, 0x7f, 0xff, 0x01, 0xff, 0xf8, 0x00, 0x00, 0x7f, 0x00, 0x00, 0x00, 0x00, 0xf7, 0xf8, 0x00, 0xff, 0x9f, 0xfe, 0x01, 0xff, 0xe0, 0x00, 0x00, 0xff, 0x00, 0x00, 0x00, 0x01, 0xfb, 0xe0, 0x00, 0x7f, 0x1f, 0xf0, 0x03, 0xff, 0xe0, 0x00, 0x00, 0x0f, 0x00, 0x00, 0x00, 0x01, 0xfd, 0x80, 0x00, 0x00, 0xff, 0xe0, 0x03, 0xff, 0xe0, 0x00, 0x01, 0xf3, 0x00, 0x00, 0x00, 0x06, 0x7f, 0x80, 0x00, 0x03, 0xff, 0xc0, 0x03, 0xff, 0xe0, 0x00, 0x03, 0xfd, 0x00, 0x00, 0x00, 0x1f, 0x1f, 0x80, 0x00, 0x07, 0xff, 0xc0, 0x00, 0x7f, 0x00, 0x00, 0x0f, 0xfe, 0x00, 0x00, 0x00, 0x3f, 0xee, 0x00, 0x00, 0x07, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xfe, 0x00, 0x00, 0x00, 0x3f, 0xf0, 0x00, 0x00, 0x0b, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0x00, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x00, 0x7c, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0x00, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x00, 0xff, 0x1e, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0x00, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xfc, 0x00, 0x00, 0x00, 0x03, 0x80, 0x00, 0x03, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, };
|
Los ejemplos para para su visualización serán los siguientes:
//Librerías SSD1306, SSD1331, Graphic VFD, PCD8544 y HX8340B display.drawBitmap(0,0,Toro,128,64,WHITE); //Librerías ST7735 e ILI9341_t3 tft.drawBitmap(0,0,Toro,128,64,0xFFFF); //Librería RGB Matrix Panel matrix.drawBitmap(0,0,Toro,128,64,matrix.Color333(0,0,0));
|
CARÁCTERES Y TEXTO CON FUENTE DE LETRA PREDETERMINADA |
Hay solo una fuente de letra predeterminada en la librería gráfica GFX de Adafruit. Cada letra de esta fuente tiene unas dimensiones de 6×8 pixels y su tamaño es escalable con multiplicadores que van de 2 a 8 (p.e. con un multiplicador de tamaño 2 tendrá unas dimensiones de 12×16 pixels). La fuente predeterminada se selecciona con la función setFont(). No es necesario seleccionarla si no hemos cambiado el tipo de letra con anterioridad.
|
void drawChar( x0, y0, carácter, color, fondo, multiplicador de tamaño) |
Para imprimir un carácter en la pantalla utilizamos la función void drawChar(), indicando las coordenadas de su esquina superior izquierda, carácter -variable tipo char-, color del carácter, color del fondo y multiplicador de tamaño. Ejemplos: //Librerías SSD1306, SSD1331, Graphic VFD, PCD8544 y HX8340B display.setFont(); display.drawChar(1,1,'a',WHITE,BLACK,1); //Color normal char caracter = 'd'; display.drawChar(9,1,caracter,BLACK,WHITE,2); //Color invertido //Librerías ST7735 e ILI9341_t3 tft.setFont(); tft.drawChar(1,1,'a',0xFFFF,0x0000,1); //Color normal char caracter = 'd'; tft.drawChar(9,1,caracter,0x0000,0xFFFF,2); //Color invertido //Librería RGB Matrix Panel matrix.setFont(); matrix.drawChar(1,1,'a',matrix.Color333(7,7,7), matrix.Color333(0,0,0),1); //Color normal char caracter = 'd'; matrix.drawChar(9,1,caracter,matrix.Color333(0,0,0), matrix.Color333(7,7,7),2); //Color invertido |
void setTextSize (multiplicador de tamaño)
void setTextColor (color de texto, color de fondo) void setCursor (x0, y0) void print (texto) o void println (texto) |
Para imprimir una cadena de texto en la pantalla utilizamos las siguientes funciones:
Ejemplos: //Librerías SSD1306, SSD1331, Graphic VFD, PCD8544 y HX8340B display.setFont(); display.setTextSize(1); //Tamaño por defecto -multiplicador 1- display.setTextColor(BLACK,WHITE); //Texto color invertido display.setCursor(1,1); display.print("aaa"); //Librerías ST7735 e ILI9341_t3 tft.setFont(); tft.setTextSize(1); tft.setTextColor(0xFFFF,0x0000); //Texto color invertido tft.setCursor(1,1); tft.print("aaa"); //Librería RGB Matrix Panel matrix.setFont(); matrix.setTextSize(1); //Texto color invertido matrix.setTextColor(matrix.Color333(7,7,7),matrix.Color333(0,0,0)); matrix.setCursor(1,1); matrix.print("aaa"); |
BORRADO O RELLENO DE LA PANTALLA |
void fillScreen(color) |
Se borra el contenido de la pantalla rellenando de un color. La función a utilizar es void fillScreen(), debiendo indicar como parámetro el color. Dependiendo del tipo de pantalla, podremos utiliza el color blanco o negro para devolverla a su estado inicial -como estaba antes de empezar a dibujar-. Ejemplos: //Librerías SSD1306 y PCD8544 display.fillScreen(0); display.drawPixel(11,10,1); display.display(); //Ejecución //Librerías SSD1331, Graphic VFD y HX8340B display.fillScreen(BLACK); display.drawPixel(11,10,WHITE); //Librerías ST7735 Y ILI9341_t3 tft.fillScreen(0x0000); tft.drawPixel(11,10,0x001F); //Librería RGB Matrix Panel matrix.fillScreen(matrix.Color333(0,0,0)); matrix.drawPixel(11,10,matrix.Color333(7,7,7)); |
REPRESENTACIÓN DE LAS FUNCIONES GRÁFICAS CON LAS LIBRERÍAS SSD1306 Y PCD8544
Para REPRESENTAR LAS FUNCIONES GRÁFICAS en la pantallas que utilizan como soporte las librerías Adafruit_SSD1306 y AdafruitPCD8544 es IMPRESCINDIBLE utilizar la instrucción display.display(). Hasta que no se ejecuta la instrucción todos los gráficos quedan almacenadas en la memoria del procesador pendientes de la orden.
Ejemplo:
//Librerías SSD1306 y PCD8544 display.fillScreen(0); display.drawPixel(17,11,1); display.display(); //Ejecución
GIRO DE LA PANTALLA
Se puede girar la posición de representación de la pantalla 90º, 180º, 270º o volver a la posición inicial (0º), medidos en el sentido de las agujas del reloj.
La función para realizar el giro es void setRotation(giro), siento el parámetro giro 0, 1, 2 ó 3 en función del giro que queramos realizar (0º, 90º, 180º ó 270º).
No podemos girar lo que ya está representado en la pantalla. El cambio solo será efectivo para las funciones gráficas que se ejecuten a partir del giro.
El origen de representación siempre estará en la esquina superior izquierda, mirando la pantalla desde el ángulo que la hayamos girado.
DIMENSIONES DE LA PANTALLA
Podemos determinar la anchura y altura de la pantalla con las funciones void width() y void height().
Teniendo en cuenta que la esquina de origen en la pantalla es la (0,0) –y no en el (1,1)-, el pixel de la esquina más alejada será el de la (anchura – 1, altura – 1).
Una vez repasado esto, estaremos listos para utilizar sin problemas la pantalla OLED.
CIRCUITO ELECTRÓNICO
El circuito que debemos conexionar es el siguiente:
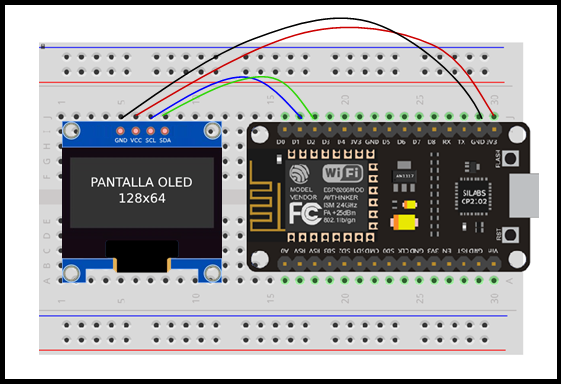
Como podemos observar,la conexión de la pantalla es simple. Dispone de cuatro puntos de conexión, dos para la alimentación (Vcc y GND) y dos para el bus de datos I2C (SCL y SDA):
PANTALLA | PLACA NodeMCU |
GND | GND |
VCC | 3V3 |
SCL | D1 (GPIO 05) |
SDA | D2 (GPIO 04) |
El bus de datos I2C admite (en teoría) la conexión de 128 dispositivos, disponiendo cada uno de ellos una dirección exclusiva de 7 bits (27 = 128). Esta dirección es simplemente un número en formato hexadecimal que permite identificar el dispositivo. En la imagen veremos un esquema de conexión I2C para entenderlo mejor.
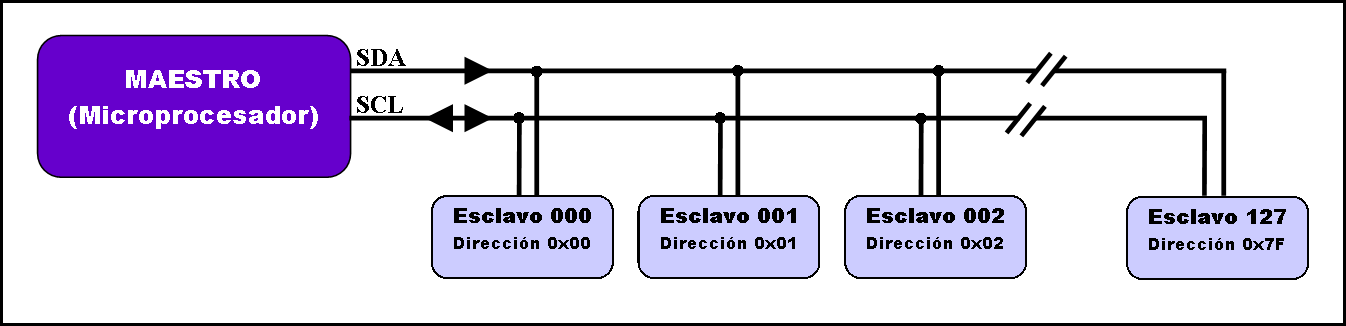
Para averiguar la dirección de conexión de la pantalla, dado que no es correlativa sino que la establece el fabricante, utilizaremos el siguiente sketch:
#include <Wire.h> void setup() { Wire.begin(); Serial.begin(9600); while (!Serial); // Espera al monitor serie Serial.println("\nEscaner I2C"); } void loop() { byte error, address; int nDevices; Serial.println("Escaneando..."); nDevices = 0; for(address = 1; address < 127; address++ ) { // El escaner i2c utiliza el valor devuelto por la instrucción // Write.endTransmisstion para reconocer la dirección a la que // está conectado cada dispositivo. Wire.beginTransmission(address); error = Wire.endTransmission(); if (error == 0) { Serial.print("Dispositivo I2C encontrado en la direccion 0x"); if (address<16) Serial.print("0"); Serial.print(address,HEX); Serial.println(" !"); nDevices++; } else if (error==4) { Serial.print("Error desconocido en la direccion 0x"); if (address<16) Serial.print("0"); Serial.println(address,HEX); } } if (nDevices == 0) Serial.println("Ningun dispositivo I2C encontrado\n"); else Serial.println("Hecho\n"); delay(5000); // espera 5 segundos para el siguiente escaneo }
El resultado será similar al siguiente:
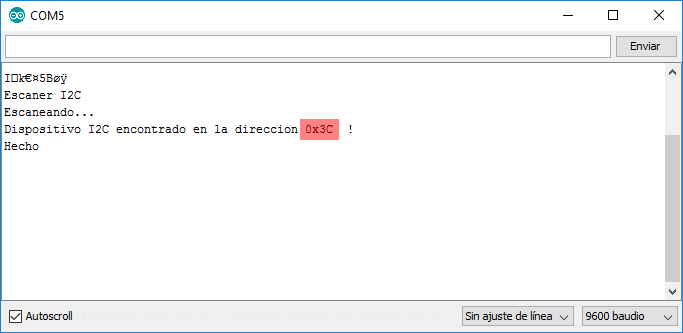
La dirección obtenida la introduciremos posteriormente en el sketch de uso de la pantalla OLED.
LIBRERÍA Y SKETCH
/* ESP8266 - Ejemplo de uso de pantalla OLED 128x64 monocroma por Dani No www.esploradores.com Este ejemplo utiliza el núcleo de la librería gráfica de Adafruit GFX y la librería SSD1306 específica para pantallas monocromas 128x64 y 128x32. Estas librerías deben estar instaladas para su funcionamiento. Para instalarlas se debe seleccionar: -Programa -> Incluir librería -> Gestionar Librerías -> Adafruit GFX Library -Programa -> Incluir librería -> Gestionar Librerías -> Adafruit SSD1306 Cuando se instala la librería SSD1306 está configurada por defecto para pantallas 128x32. Al utilizarla para una pantalla 128x64 es preciso reconfigurarla. Para hacerlo debe abrir el fichero Adafruit_SSD1306.h con un editor y descomentar la línea 69 (// #define SSD1306_128_64 ---> #define SSD1306_128_64) y comentar la línea 70 (#define SSD1306_128_32 ---> //#define SSD1306_128_32). Así mismo se debe verificar en la línea 51 que la dirección de I2C de la pantalla es correcta (#define SSD1306_I2C_ADDRESS 0x3C // 128x64 0x3D (default) or 0x3C (if SA0 is grounded)) Por último, también se debe verificar que en la línea 108 de este sketch la dirección I2C de la pantalla también es correcta. */ #include <SPI.h> #include <Wire.h> #include <Adafruit_GFX.h> //Nucleo de la librería gráfica. #include <Adafruit_SSD1306.h> //Librería para pantallas OLED monocromas de 128x64 y 128x32 #include <Fonts/FreeMonoBoldOblique12pt7b.h> #include <Fonts/FreeSansBoldOblique12pt7b.h> #define OLED_RESET 0 Adafruit_SSD1306 display(OLED_RESET); #define NUMFLAKES 10 #define XPOS 0 #define YPOS 1 #define DELTAY 2 const unsigned char Toro [] PROGMEM = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7f, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0xff, 0xff, 0xe0, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x70, 0x00, 0x00, 0x00, 0x0f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x80, 0x00, 0x00, 0x18, 0x00, 0x00, 0x00, 0x1c, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x18, 0x00, 0x00, 0x00, 0x7d, 0xbf, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x18, 0x00, 0x00, 0x00, 0xfd, 0xbf, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x1c, 0x00, 0x00, 0x00, 0xfd, 0xdf, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x1c, 0x00, 0x00, 0x07, 0xfd, 0xdf, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x1c, 0x00, 0x00, 0x0f, 0xfd, 0xdf, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x1c, 0x00, 0x00, 0x0f, 0xff, 0xef, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf4, 0x00, 0x00, 0x1e, 0x00, 0x00, 0x3f, 0xff, 0xef, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf7, 0xc0, 0x00, 0x1e, 0x00, 0x00, 0x3f, 0xfb, 0xef, 0xff, 0xf3, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf7, 0xf8, 0x00, 0x1e, 0x00, 0x00, 0xff, 0xfb, 0xef, 0xff, 0xfb, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf7, 0xfc, 0x00, 0x1f, 0xe1, 0xfe, 0x7f, 0xfb, 0xef, 0xff, 0xfd, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf7, 0xc4, 0x00, 0x1f, 0xff, 0xff, 0xbf, 0xfb, 0xef, 0xff, 0xfd, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf0, 0x7c, 0x00, 0x1f, 0xff, 0xff, 0xc7, 0xf7, 0xef, 0xff, 0xfe, 0xff, 0xff, 0xff, 0xbf, 0xff, 0xf7, 0xe4, 0x00, 0x0f, 0xff, 0xff, 0xf9, 0xe7, 0xef, 0xff, 0xfe, 0xff, 0xff, 0xff, 0xbf, 0xff, 0xf7, 0xfc, 0x00, 0x07, 0xff, 0xff, 0xfc, 0x1f, 0xdf, 0xff, 0xfe, 0xff, 0xff, 0xff, 0xbf, 0xff, 0xef, 0xdc, 0x00, 0x03, 0xbf, 0xff, 0xfb, 0xff, 0xc7, 0xff, 0xff, 0x7f, 0xff, 0xff, 0xbf, 0xff, 0xef, 0xbc, 0x00, 0x01, 0xbf, 0xff, 0xff, 0xff, 0xb7, 0xff, 0xff, 0x7f, 0xff, 0xff, 0xbf, 0xff, 0xef, 0x3c, 0x00, 0x00, 0x3f, 0xff, 0xff, 0xfe, 0x77, 0xff, 0xff, 0x7f, 0xff, 0xff, 0xbf, 0xff, 0xef, 0x3c, 0x00, 0x01, 0xff, 0xff, 0xff, 0xfd, 0xf7, 0xff, 0xff, 0x7f, 0xff, 0xff, 0xbf, 0xff, 0xef, 0x3c, 0x00, 0x03, 0xff, 0xff, 0xff, 0xfb, 0xef, 0xff, 0xff, 0x7f, 0xff, 0xff, 0x7f, 0xff, 0xf7, 0x3c, 0x00, 0x03, 0xff, 0xff, 0xff, 0xe7, 0xcf, 0xff, 0xff, 0x7f, 0xff, 0xff, 0x7f, 0xff, 0xf7, 0x3c, 0x00, 0x03, 0xff, 0xff, 0xff, 0x1f, 0xdf, 0xff, 0xff, 0x7f, 0xff, 0xff, 0x7f, 0xff, 0xf7, 0xbc, 0x00, 0x03, 0xff, 0xff, 0xff, 0xff, 0x9f, 0xff, 0xff, 0x7f, 0xff, 0xff, 0x7f, 0xff, 0xe3, 0xdc, 0x00, 0x03, 0xff, 0xff, 0xff, 0xfe, 0x3f, 0xfc, 0xff, 0x7f, 0xff, 0xfe, 0xff, 0xff, 0xe1, 0xe4, 0x00, 0x03, 0xff, 0xfc, 0xff, 0x8c, 0x7f, 0xf1, 0x3f, 0x7f, 0xff, 0xfe, 0xff, 0xff, 0xc1, 0xfc, 0x00, 0x01, 0xff, 0xf0, 0xff, 0x81, 0xff, 0xff, 0xcf, 0xff, 0xff, 0xff, 0xff, 0xff, 0xc0, 0xfe, 0x00, 0x01, 0xff, 0xf3, 0xff, 0x9f, 0xff, 0xff, 0xcf, 0xff, 0xff, 0xef, 0xff, 0xff, 0x80, 0x7e, 0x00, 0x03, 0xff, 0xff, 0xff, 0x9f, 0xff, 0xff, 0xf6, 0xff, 0xff, 0xdf, 0xff, 0xff, 0x80, 0x3e, 0x00, 0x03, 0xff, 0xff, 0xff, 0x9f, 0xff, 0xff, 0xf7, 0xff, 0xff, 0xbf, 0xff, 0xfe, 0x00, 0x1c, 0x00, 0x03, 0xff, 0xff, 0xff, 0x9f, 0xff, 0xff, 0xf7, 0xff, 0xff, 0x7f, 0x7f, 0xfe, 0x00, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0x1f, 0xff, 0xff, 0xf7, 0xff, 0xf8, 0xff, 0x3f, 0xfc, 0x00, 0x00, 0x00, 0x07, 0xff, 0xff, 0xff, 0x7f, 0xff, 0xff, 0xf7, 0xff, 0xe7, 0x7e, 0x0f, 0xfc, 0x00, 0x00, 0x00, 0x1f, 0xff, 0xff, 0xff, 0x7f, 0xff, 0xff, 0xf7, 0xff, 0xcf, 0x80, 0x07, 0xfc, 0x00, 0x00, 0x00, 0x7f, 0x9f, 0xff, 0xfe, 0x7f, 0xff, 0xff, 0xf7, 0xfc, 0x3f, 0xe0, 0x07, 0xfc, 0x00, 0x00, 0x00, 0x7e, 0x37, 0xff, 0xfe, 0xff, 0xff, 0xff, 0xcf, 0xe2, 0x7f, 0x80, 0x07, 0xfc, 0x00, 0x00, 0x00, 0xfe, 0x7e, 0x1f, 0xfd, 0xff, 0xff, 0xff, 0xb8, 0x1d, 0xfe, 0x00, 0x0f, 0xfc, 0x00, 0x00, 0x00, 0xfe, 0x3f, 0xdf, 0xf9, 0xff, 0xff, 0xff, 0x77, 0xfb, 0xf8, 0x00, 0x0f, 0xf8, 0x00, 0x00, 0x00, 0x7f, 0xdf, 0xef, 0xf3, 0xff, 0xff, 0xfe, 0xff, 0xf7, 0xe0, 0x00, 0x0f, 0xf8, 0x00, 0x00, 0x00, 0x7f, 0xfe, 0x6f, 0xc7, 0xff, 0xff, 0xf0, 0x7f, 0xef, 0xc0, 0x00, 0x0f, 0xf0, 0x00, 0x00, 0x00, 0x7f, 0xf9, 0xef, 0x8f, 0xff, 0xff, 0xe0, 0x3f, 0xee, 0x00, 0x00, 0x0f, 0xe0, 0x00, 0x00, 0x00, 0x0f, 0x87, 0xee, 0x7d, 0xff, 0xff, 0xc0, 0x0f, 0x9c, 0x00, 0x00, 0x3f, 0x80, 0x00, 0x00, 0x00, 0x0f, 0xff, 0x00, 0xfe, 0xff, 0xff, 0x80, 0x7f, 0x7c, 0x00, 0x00, 0x3f, 0x80, 0x00, 0x00, 0x00, 0x68, 0x3e, 0x00, 0xff, 0x7f, 0xff, 0x01, 0xff, 0xf8, 0x00, 0x00, 0x7f, 0x00, 0x00, 0x00, 0x00, 0xf7, 0xf8, 0x00, 0xff, 0x9f, 0xfe, 0x01, 0xff, 0xe0, 0x00, 0x00, 0xff, 0x00, 0x00, 0x00, 0x01, 0xfb, 0xe0, 0x00, 0x7f, 0x1f, 0xf0, 0x03, 0xff, 0xe0, 0x00, 0x00, 0x0f, 0x00, 0x00, 0x00, 0x01, 0xfd, 0x80, 0x00, 0x00, 0xff, 0xe0, 0x03, 0xff, 0xe0, 0x00, 0x01, 0xf3, 0x00, 0x00, 0x00, 0x06, 0x7f, 0x80, 0x00, 0x03, 0xff, 0xc0, 0x03, 0xff, 0xe0, 0x00, 0x03, 0xfd, 0x00, 0x00, 0x00, 0x1f, 0x1f, 0x80, 0x00, 0x07, 0xff, 0xc0, 0x00, 0x7f, 0x00, 0x00, 0x0f, 0xfe, 0x00, 0x00, 0x00, 0x3f, 0xee, 0x00, 0x00, 0x07, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x0f, 0xfe, 0x00, 0x00, 0x00, 0x3f, 0xf0, 0x00, 0x00, 0x0b, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0x00, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x00, 0x7c, 0xff, 0x80, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0x00, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x00, 0xff, 0x1e, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1f, 0xff, 0x00, 0x00, 0x00, 0x7f, 0xf8, 0x00, 0x01, 0xff, 0xe0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xfc, 0x00, 0x00, 0x00, 0x03, 0x80, 0x00, 0x03, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xf8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xff, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, }; void setup() { display.begin(SSD1306_SWITCHCAPVCC, 0x3C); // //Inicializa pantalla con en la dirección 0x3D para la conexión I2C. } void loop() { //PUNTOS EN LAS CUATRO ESQUINAS DE LA PANTALLA display.fillScreen(0); //Limpiamos la pantalla display.drawPixel(0,0,1); //Esquina superior izquierda display.drawPixel(127,0,1); //Esquina superior derecha display.drawPixel(0,63,1); //Esquina inferior izquierda display.drawPixel(127,63,1); //Esquina inferior derecha display.display(); //Refrescamos la pantalla para visualizarlos delay(4000); //LÍNEAS CRUZADAS DE ESQUINA A ESQUINA display.fillScreen(0); //Limpiamos la pantalla display.drawLine(0,0,127,63,1); display.drawLine(0,display.height()-1,display.width()-1,0,1); display.display(); //Refrescamos la pantalla para visulizarlas delay(4000); //TRIÁNGULO Y TRIÁNGULO RELLENO display.fillScreen(0); //Limpiamos la pantalla display.drawTriangle(0,0,127,0,64,32,1); display.fillTriangle(0,display.height()-1,display.width()-1,display.height()-1,display.width()/2,display.height()/2,1); display.display(); //Refrescamos la pantalla para visualizarlos delay(4000); //RECTÁNGULO Y RECTÁNGULO RELLENO display.fillScreen(0); //Limpiamos la pantalla display.drawRect(0,0,127,31,1); display.fillRect(0,display.height()/2+1,display.width()-1,display.height()/2-1,1); display.display(); //Refrescamos la pantalla para visualizarlos delay(4000); //RECTÁNGULO REDONDEADO Y RECTÁNGULO REDONDEADO RELLENO display.fillScreen(0); //Limpiamos la pantalla display.drawRoundRect(0,0,127,31,10,1); display.fillRoundRect(0,display.height()/2+1,display.width()-1,display.height()/2-1,10,1); display.display(); //Refrescamos la pantalla para visualizarlos delay(4000); //CÍRCULO Y CÍRCULO RELLENO display.fillScreen(0); //Limpiamos la pantalla display.drawCircle(32,32,30,1); display.fillCircle(display.width()*3/4,display.height()/2,30,1); display.display(); //Refrescamos la pantalla para visualizarlos delay(4000); //CARÁCTERES CON FUENTE PREDETERMINADA display.fillScreen(0); //Limpiamos la pantalla display.setFont(); //Fuente por defecto -si no la hemos cambiado no es necesario seleccionarla display.drawChar(8,0,'a',1,0,8); //Tamaños de 1 a 8. Los tamaños típicos son 1, 2 o 4 char caracter1 = 'd'; display.drawChar(65,0,caracter1,0,1,8); display.display(); //Refrescamos la pantalla para visualizarlos delay(4000); //TEXTO CON FUENTE PREDETERMINADA display.fillScreen(0); //Limpiamos la pantalla display.setFont(); //Fuente por defecto -si no la hemos cambiado no es necesario seleccionarla display.setTextSize(1); display.setTextColor(1,0); display.setCursor(0,0); display.println("AaBbCcDdEeFfGgHhIiJjK"); display.setTextSize(2); display.print("AaBbCcDdEe"); display.setTextSize(3); display.setTextColor(0,1); //Color invertido display.setCursor(0,32); display.print("AaBbCcD"); display.display(); //Refrescamos la pantalla para visualizarlo delay(4000); //GIRO DE PANTALLA -La coordenada (0,0) siempre queda en la esquina superior izquierda //display.setRotation(0); //0 grados -posición inicial- //display.setRotation(1); //90 grados en el sentido de las agujas del reloj //display.setRotation(2); //180 grados en el sentido de las agujas del reloj display.setRotation(3); //270 grados en el sentido de las agujas del reloj //CARÁCTER CON FUENTE ALTERNATIVA display.fillScreen(0); //Limpiamos la pantalla display.setFont(&FreeMonoBoldOblique12pt7b); //Fuente FreeSansBoldOblique12pt7b display.drawChar(0,85,'A',1,0,4); display.display(); //Refrescamos la pantalla para visualizarlo delay(4000); //TEXTO CON FUENTES ALTERNATIVAS display.fillScreen(0); //Limpiamos la pantalla display.setFont(&FreeMonoBoldOblique12pt7b); //Fuente FreeMonoBoldOblique12pt7b display.setTextSize(1); display.setTextColor(1); display.setCursor(0,20); display.println("AaBb"); display.setTextSize(2); display.setCursor(0,53); display.println("Aa"); display.setFont(&FreeSansBoldOblique12pt7b); //Fuente FreeSansBoldOblique12pt7b display.setTextSize(1); display.setTextColor(1); display.setCursor(0,85); display.println("AaBb"); display.setTextSize(2); display.setCursor(0,127); display.println("Aa"); display.display(); //Refrescamos la pantalla para visualizarlos delay(4000); //GIRO DE PANTALLA A LA POSICIÓN INICIAL display.setRotation(0); //IMAGEN (TORO) display.fillScreen(0); //Limpiamos la pantalla display.drawBitmap(0,0,Toro,128,64,1); display.display(); //Refrescamos la pantalla para visualizarla delay(4000); }
Una vez subido el sketch a la placa se visualizarán diréctamente los gráficos en la pantalla.
Leave a Reply
Tu correo electrónico está seguro.
You must be logged in to post a comment.